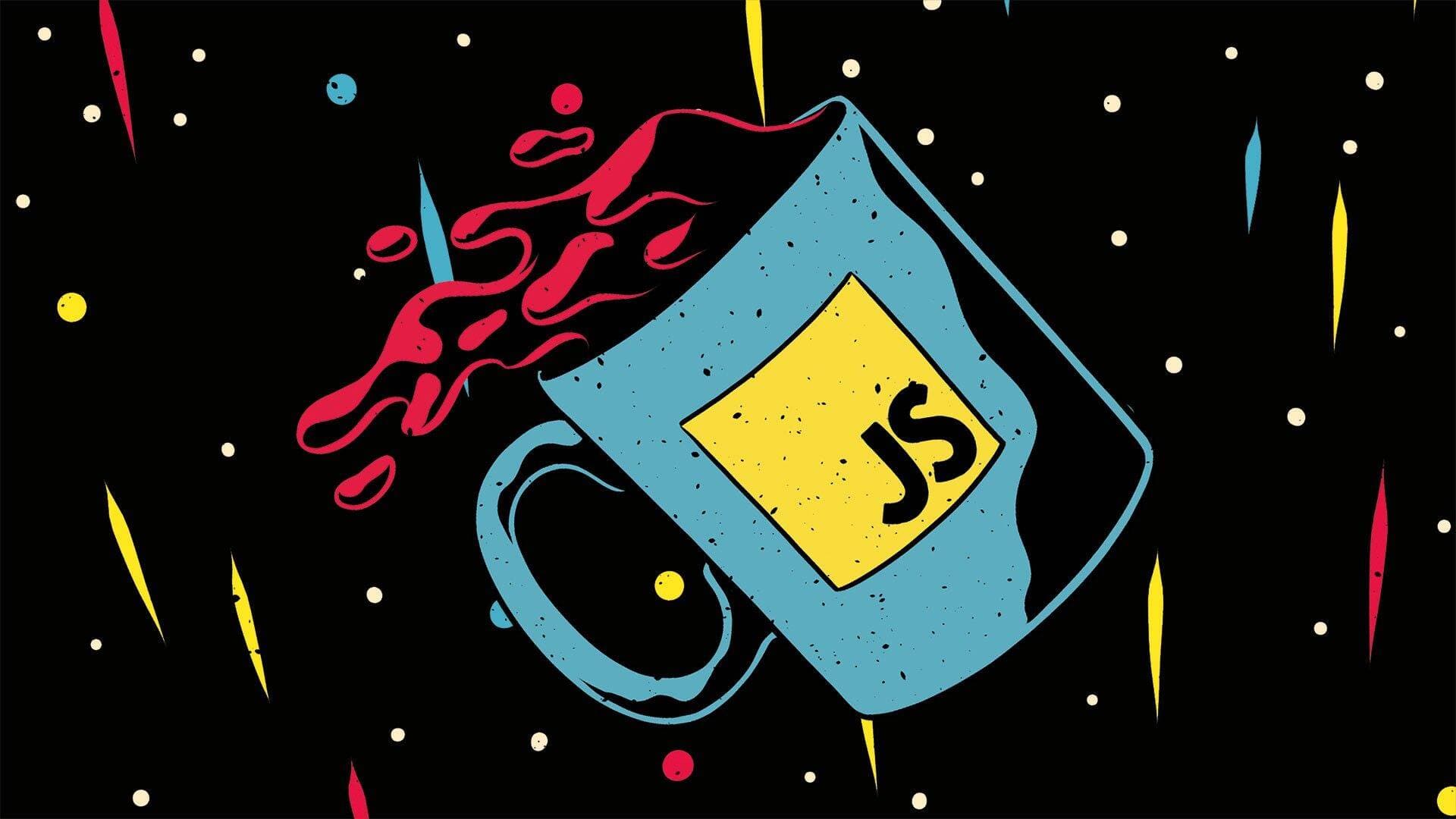
Why You Can Use Method In Primitive Types?
JavaScript, being a dynamic and weakly-typed language, allows variables to change types dynamically at runtime. While primitive types in JavaScript (such as numbers, strings, booleans, null
, undefined
, and Symbol
) are not objects and thus do not have methods or properties, we can still call methods and access properties on them.
This is made possible by the mechanisms of boxing and unboxing.
Boxing is the process of converting a primitive type to its corresponding object type. JavaScript provides three object constructors for boxing:
Number
for numeric values.String
for string values.Boolean
for boolean values.
When you call a method or access a property on a primitive type, JavaScript automatically boxes the primitive value into its corresponding object type.
This boxing is temporary, existing only for the duration of the method call or property access.
let a = 42;
console.log(a.toString()); // "42"
In this example, a
is a number, but we call the toString()
method on it. The JavaScript engine performs the following steps:
- Creates a
Number
object that wraps the value ofa
. - Calls the
toString()
method on theNumber
object. - Destroys the temporary
Number
object and returns the string"42"
.
Unboxing is the process of converting an object type back to its corresponding primitive type.
When you attempt to perform an operation that requires a primitive type on an object, JavaScript automatically unboxes the object.
let b = new Number(42);
let c = b + 8; // 50
In this example, b
is a Number
object. When we perform the addition operation, JavaScript does the following:
- Unboxes the
b
object to its primitive value42
. - Performs the addition
42 + 8
, resulting in50
.
Note:
Boxing and unboxing are implemented at runtime by the JavaScript engine (e.g., V8 in Node.js and Chrome). When the code is executed, the engine dynamically handles the conversion between primitive types and their corresponding object types.
This process is not part of the AST (Abstract Syntax Tree) generation or parsing phase but occurs during the actual execution of the code.
-
Performance Issues: Frequent boxing and unboxing can impact performance because each operation requires the creation and destruction of temporary objects. Avoid unnecessary boxing and unboxing when possible.
-
Comparison Operations: Comparisons between boxed objects and primitive types can yield unexpected results. For example:
let d = new Number(42); console.log(d == 42); // true console.log(d === 42); // false
In the first comparison, the
==
operator performs type conversion, resulting intrue
.In the second comparison, the
===
operator does not perform type conversion, resulting infalse
.
These mechanisms allow us to call methods and access properties on primitive types, enhancing code flexibility and readability. However, we must be mindful of their performance implications and the potential for unexpected results in comparison operations.
Key Takeaway: Boxing and unboxing are runtime behaviors implemented by the JavaScript engine. They enable primitive types to temporarily behave like objects, but this flexibility comes with a performance cost that developers should be aware of.