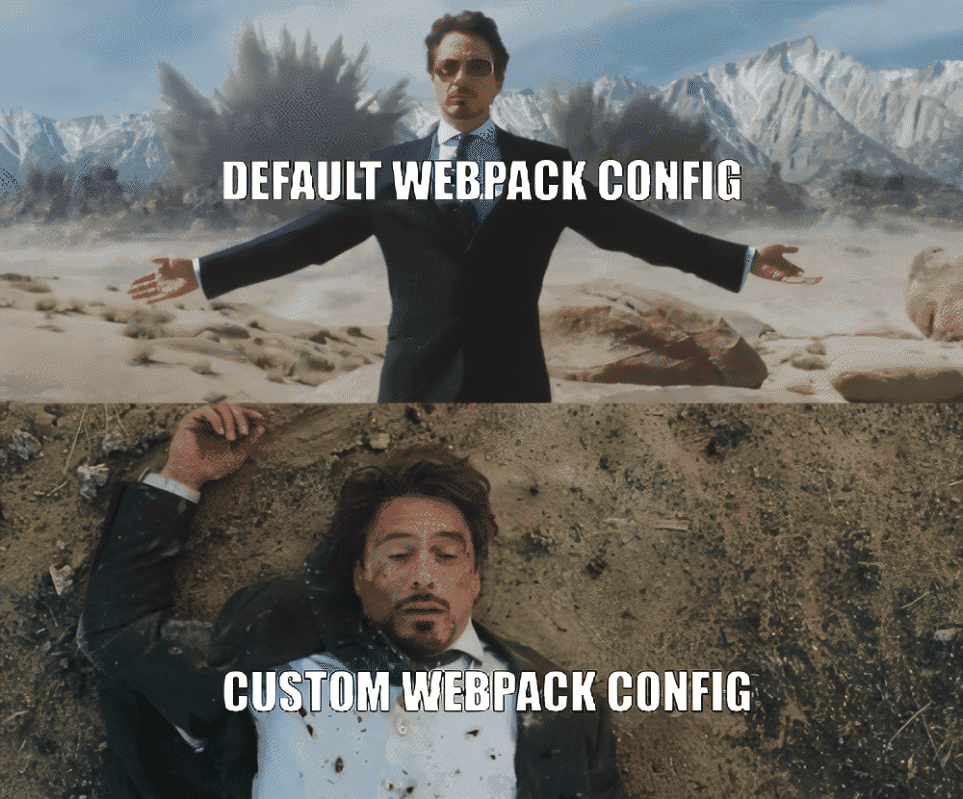
Uncommon Webpack Bundle Performance Optimization: Externals
Webpack is a familiar tool for many. It is a module bundler that treats various resources in an application (like JavaScript, CSS, images, etc.) as modules and bundles them into one or more files.
If you are new to Webpack, I recommend reading this article first:
In this article, I will introduce you to a special Webpack configuration: externals
.
According to the official documentation, if we want to use a library but do not want Webpack to bundle it, we can configure externals
. This allows us to use the library in CMD, AMD, or global variable (like window
) formats.
For example, suppose we are developing our own library and we want to include Lodash. When we bundle with Webpack, we notice that including Lodash increases the file size significantly. In this case, we can use externals
to reference Lodash, meaning our library will not bundle Lodash but will expect the user to provide it in their environment.
import _ from 'lodash';
externals: {
"lodash": {
commonjs: "lodash", // In Node.js, import _ from 'lodash' is equivalent to const _ = require('lodash');
commonjs2: "lodash", // Same as above
amd: "lodash", // If using require.js, it is equivalent to define(["lodash"], factory);
root: "_" // In the browser, a global variable '_' must be provided, equivalent to var _ = (window._) || (_);
}
}
In summary, the purpose of the externals
configuration is to allow import _ from 'lodash'
to work correctly in various environments without including Lodash in the bundle.
It's important to note that if Lodash does not provide _
as a global variable in the browser, you will not be able to use it. The variable "_"
cannot be changed arbitrarily. If the external library provides a global variable lodash
, you should use lodash
.
If your library needs to support multiple environments, it is recommended to set libraryTarget
to umd
in the output
. This way, the bundled file will comply with the UMD specification, making it suitable for various environments. libraryTarget
and externals
are closely related.
In modern front-end development, performance optimization is crucial for improving user experience. Using externals
can significantly reduce the size of the bundled file, thus enhancing loading speed and application performance. Here are some strategies and examples of how to use externals
for performance optimization.
By marking commonly used libraries (like Lodash, jQuery, etc.) as externals, we can avoid bundling them into our application. This is especially important for large libraries, which can take up a lot of space.
For example, if your application relies on Lodash but there is already a global Lodash included, you can use the following configuration to avoid bundling it again:
externals: {
"lodash": "_"
}
With this configuration, Webpack will not include Lodash in your files, expecting to find the global variable _
in the runtime environment.
Using a CDN to serve libraries not only reduces build time but also leverages the CDN's caching mechanism to speed up resource loading. You can simply add a <script>
tag in your HTML file, like this:
<script src="https://cdn.jsdelivr.net/npm/lodash/lodash.min.js"></script>
We already configured Webpack to use externals
to tell it not to bundle Lodash:
externals: {
"lodash": "_"
}
This ensures that your application loads resources from the CDN, improving loading speed.
Setting less frequently changed or used libraries as externals can reduce the build time for each build. Webpack will not need to analyze and bundle these libraries, speeding up the overall build process.
By configuring externals
properly, we can effectively reduce the size of the bundled file, utilize CDNs to accelerate resource loading, adapt to multiple environments, and decrease build time. These strategies not only enhance application performance but also improve user experience. In actual development, it is advisable to use externals
flexibly based on project needs to achieve optimal performance optimization.