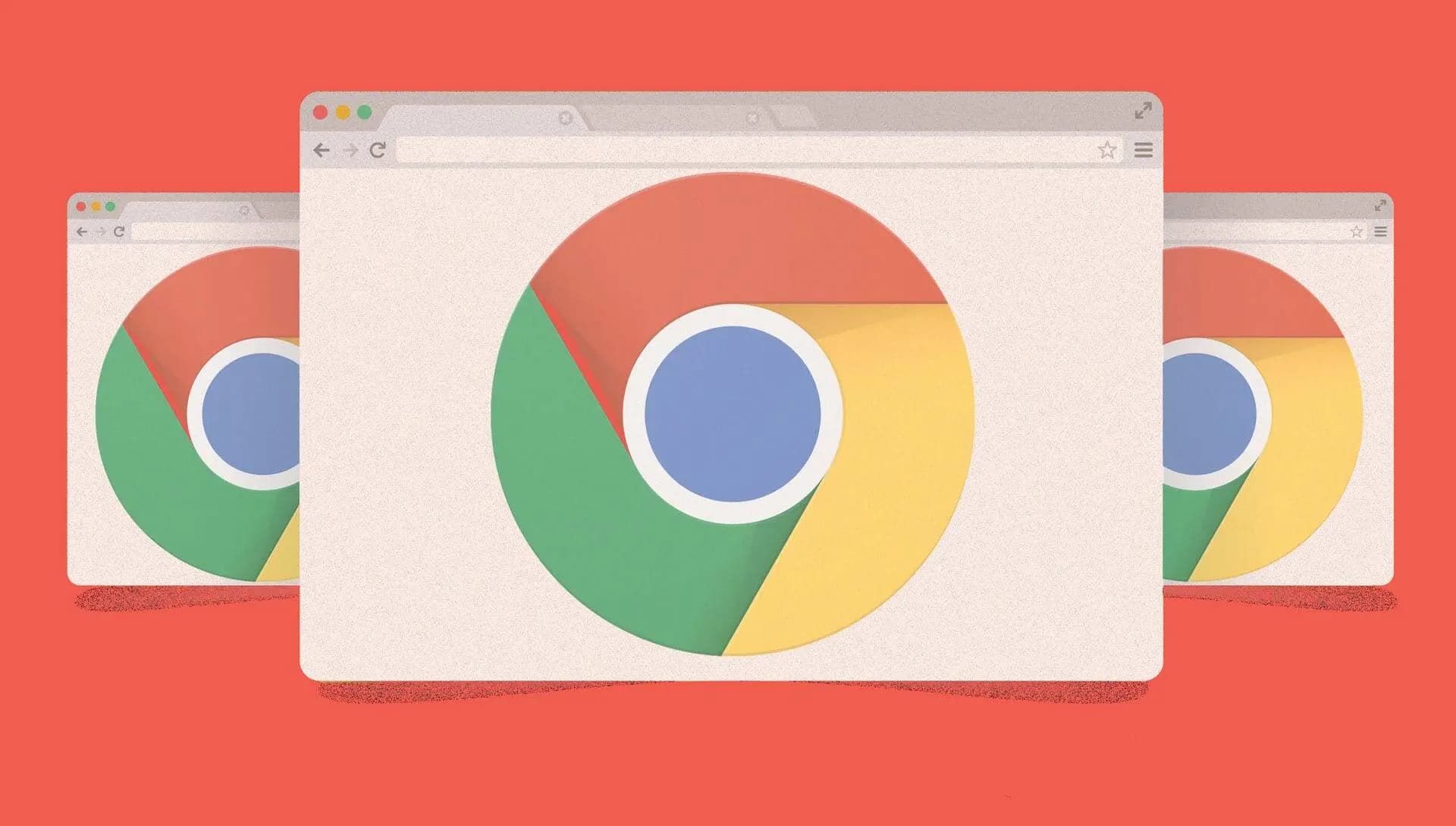
Create a Chrome Extension Using Vite & React
The Chrome browser extension (commonly referred to as CRX) is already well-known among developers. Current Chrome Extension development must adhere to the Manifest V3 specification. According to Google's official announcement, as of June 2023, publishing Chrome Extensions using Manifest V2 is no longer permitted, and all Manifest V2 extensions are expected to be completely removed by 2024.
As Manifest V2 approaches its end, this series of tutorials will focus exclusively on Manifest V3 (abbreviated as MV3) content.
In recent times, many front-end developers have transitioned to using Vite, which motivated the creation of this tutorial. It aims to provide a comprehensive guide on developing Chrome extensions with Vite, helping you save time, avoid unnecessary detours, and expedite your project development.
This tutorial document is extensive, so it’s advisable to review the table of contents to familiarize yourself with the topics covered.
We recommend following the chapters in order as you learn and practice. This structured approach will help you quickly grasp the principles and nuances of the entire project. In the future, when you encounter new challenges, you’ll have a solid foundation to build upon.
Creating a Google Chrome extension with Plasmo can be relatively straightforward. However, in this guide, we will not rely on frameworks for development. Instead, we will use tools like Vite to systematically construct our plugin framework from the ground up.
I won’t delve into basic introductions, as I believe most of you have already used Chrome extensions or have a basic understanding of them.
If you know nothing about Chrome extensions, check here create your Chrome extension.
Since you’re ready to read on, you already possess some fundamental knowledge. Below, I will outline three key questions that are of utmost importance:
-
What are Extensions?: Chrome extensions enhance the browsing experience by customizing the user interface, monitoring browser events, and modifying web content.
-
How are They Built?: Extensions are built using the same web technologies employed in creating web applications: HTML, CSS, and JavaScript.
-
What Can They Do?: Apart from utilizing Web APIs, extensions also have access to Chrome Extension APIs, allowing them to perform various tasks.
When developing Chrome extensions with Vite, CRXJS—a Vite plugin—often comes up in discussions within the tech community.
However, this tutorial chooses not to use CRXJS for several reasons:
-
As of this writing, the CRXJS website only offers a Beta version compatible with Vite 3, while Vite has already progressed to version 5.
-
It's generally more reliable to utilize core functionalities from official sources whenever possible. By modifying the official architecture as needed, developers can take control of their projects, resolve issues independently, and gain a deeper understanding of the underlying technologies.
-
The more external dependencies a project relies on, the greater the learning curve and maintenance costs become.
Alright, let's get started.
Given that the LTS version of Node.js has been upgraded to 20, it is recommended to use the latest version of Node.js to ensure that Vite runs properly.
First, navigate to the directory where you want to create your project. Then, execute the installation command.
I recommend using the package manager pnpm. Therefore, the command you need to enter is:
$ pnpm create vite@latest
After execution, the system will prompt you to enter a project name. I entered "vite-react-extension," but feel free to customize it as needed.
Project name: vite-react-extension
Next, you will be asked to choose a framework. Please select "React":
Select a framework:
Vanilla
Vue
❯ React
Preact
Lit
Svelte
Solid
Qwik
Others
Finally, choose the development language. For this tutorial, we will select TypeScript + SWC:
Select a variant:
TypeScript
> TypeScript + SWC
JavaScript
JavaScript + SWC
After answering these "three soulful questions," your Vite project creation will be complete.
If you haven't installed pnpm yet, you can do so globally by executing the following command:
npm install --global pnpm
Sure! Here’s your text formatted and translated into English:
-
Navigate to the Project Directory
Enter the project directory and run the command to install the project dependencies:cd vite-react-extension
-
Install Dependencies
Run the following command to install the dependencies:pnpm install
After a moment, the installation will be complete.
-
Run the Project
Execute the following command to run the project:pnpm run dev
You may notice that the compilation speed is very fast, almost instantaneous.
-
Access the Project
Now, you need to manually open the following address to access the project:http://localhost:5173/
When everything is ready, you should see the following picture in your browser:
Next, delete any unnecessary files to simplify the project structure.
The original project structure is as follows:
├─ /node_modules
├─ /public
│ └─ vite.svg
├─ /src
│ ├─ /assets
│ │ └─ react.svg
│ ├─ App.css
│ ├─ App.tsx
│ ├─ index.css
│ ├─ vite-env.d.ts
│ └─ main.tsx
├─ .eslintrc.cjs
├─ .gitignore
├─ index.html
├─ package.json
├─ tsconfig.app.json
├─ tsconfig.node.json
├─ tsconfig.json
├─ README.md
├─ vite.config.ts
└─ pnpm-lock.yaml
We will adjust it to the following structure:
├─ /node_modules
├─ /public
├─ /src
│ ├─ App.tsx
│ ├─ vite-env.d.ts
│ └─ main.tsx
├─ .eslintrc.cjs
├─ .gitignore
├─ index.html
├─ package.json
├─ tsconfig.app.json
├─ tsconfig.node.json
├─ tsconfig.json
├─ README.md
├─ vite.config.ts
└─ pnpm-lock.yaml
Now it looks cleaner, but deleting the above files will cause errors on the page. This is because the corresponding file references no longer exist. We need to continue modifying the code to ensure the project runs properly.
Modify the following files one by one to streamline the code as follows:
src/App.tsx:
export function App() {
return <>Hello Cool Boiled Water</>;
}
src/main.tsx:
import { StrictMode } from 'react';
import { createRoot } from 'react-dom/client';
import { App } from './App.tsx';
createRoot(document.getElementById('root')!).render(
<StrictMode>
<App />
</StrictMode>
);
index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Vite-React-Extension</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/src/main.tsx"></script>
</body>
</html>
With these changes, your code should run correctly. If it doesn't, use your problem-solving skills to get the project up and running.