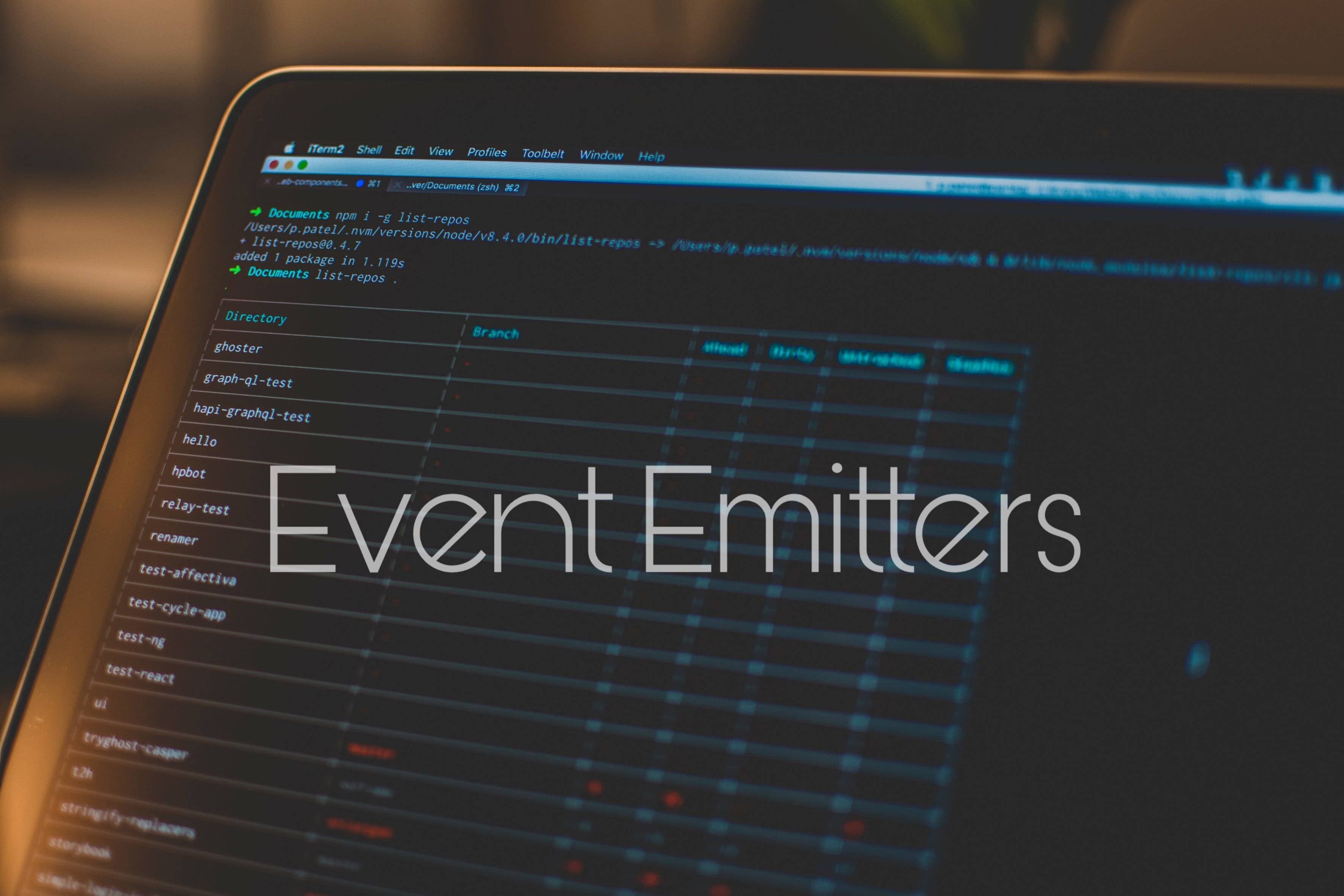
Browser Event Mechanisms: EventTarget vs EventEmitter
Event handling is a crucial task in frontend development. JavaScript offers various mechanisms for event handling, among which two common approaches are through the EventTarget
interface in browsers and the EventEmitter
class in Node.js. This article will delve into the principles, usage, and pros and cons of these two mechanisms.
The EventTarget
interface is a standard event handling mechanism provided by browsers. It defines a set of methods for adding, removing, and triggering event listeners on nodes in the DOM tree. Almost all DOM elements implement the EventTarget
interface, including the document object (Document
), element objects (Element
), document fragment objects (DocumentFragment
), etc. Within the DOM standard, there are three main methods:
addEventListener(type, listener[, options])
: Adds an event listener to the event target.removeEventListener(type, listener[, options])
: Removes an event listener from the event target.dispatchEvent(event)
: Manually triggers an event of the specified type.
Sometimes, when you delve into the browser's event loop, you might wonder about the relationship between the EventTarget
, which follows the observer pattern, and the task queue of the event loop. The answer is simple: when an event is triggered on an EventTarget
, all callback functions for that event are pushed into the macro task queue, awaiting execution.
Let's elaborate on the detailed process:
-
Registration of Event Listeners:
- Event listeners are added to DOM elements either through the
addEventListener
method or through helper methods provided by browsers for simplification, such asonClick
.
- Event listeners are added to DOM elements either through the
-
Event Triggering:
- When a specific event occurs in the browser (e.g., click event, keyboard event, etc.), the browser first determines the affected element.
- The browser creates an event object based on the event type and target element and sets the corresponding properties (e.g.,
type
,target
, etc.).
-
Event Propagation:
- Event propagation consists of three phases: capturing phase, target phase, and bubbling phase.
- In the capturing phase, the event propagates from the document root to the target element, traversing parent elements until reaching the target.
- In the target phase, the event reaches the target element and triggers the event handlers.
- In the bubbling phase, the event propagates from the target element back to the document root, traversing parent elements.
-
Event Handling:
- During event propagation, the browser checks each traversed element for event listeners of the corresponding type. It's worth noting that a DOM object can have event listeners for both capturing and bubbling phases, and by default, listeners added via
addEventListener
are for the bubbling phase. - If an event listener is found, the browser calls the registered event handler and passes the corresponding event object as a parameter.
- These event handlers can access properties of the event object. It's generally recommended to use
event.currentTarget
to obtain more information about the event and execute the appropriate logic.
- During event propagation, the browser checks each traversed element for event listeners of the corresponding type. It's worth noting that a DOM object can have event listeners for both capturing and bubbling phases, and by default, listeners added via
-
Event Loop:
- When an event is triggered, the registered listeners are added to the macro task queue.
- When the execution stack is empty and there are no pending microtasks, the event loop dequeues a task from the macro task queue for execution. Thus, if a click action is bound to multiple listeners, it may execute across multiple ticks.
In summary, EventTarget
is an interface for adding event listeners to DOM elements. Its relationship with the browser event loop lies in the fact that registered event listeners are ultimately added to the task queue of the event loop and executed at specific times.
EventEmitter
is a commonly used event-driven programming mechanism in Node.js, implementing custom event management through the observer pattern. Although EventEmitter
is provided by Node.js, it can also be used in browsers through libraries like eventemitter3
. Its main functionalities include:
- Registering event listeners: Adding event listeners through methods like
on()
oraddListener()
. - Triggering events: Emitting events using the
emit()
method and executing corresponding listeners. - Removing event listeners: Removing specific event listeners through methods like
off()
orremoveListener()
.
EventEmitter
provides a flexible and powerful event handling mechanism, allowing developers to conveniently manage and handle events.
Below is how you can use EventEmitter natively in Node.js:
import events from 'events';
const eventEmitter = new events.EventEmitter();
However, if you want to use an EventEmitter-like mechanism in the frontend, such as implementing an event bus for scenarios where updates in one component require simultaneous responses from other distant components, traditional methods like React's event propagation or global variables might not be suitable. Therefore, you would either need to implement your own event bus or use third-party libraries.
Let's attempt to implement an EventEmitter ourselves and understand its underlying principles. Once we grasp the concept, we'll be better equipped to utilize it in the future.
class EventEmitter {
constructor() {
// Initialize an empty object to store event listeners
this.events = {};
}
// Method to add an event listener for a specific event
on(eventName, listener) {
// If there are no listeners for this event yet, create an array to store them
if (!this.events[eventName]) {
this.events[eventName] = [];
}
// Add the listener function to the array of listeners for the specified event
this.events[eventName].push(listener);
}
// Method to emit (trigger) an event and call all its listeners
emit(eventName, ...args) {
// Get the array of listeners for the specified event
const listeners = this.events[eventName];
// If there are listeners for this event, call each listener function with provided arguments
if (listeners) {
listeners.forEach(listener => {
listener(...args);
});
}
}
// Method to remove a specific listener for a given event
off(eventName, listenerToRemove) {
// Get the array of listeners for the specified event
const listeners = this.events[eventName];
// If there are listeners for this event, filter out the listenerToRemove
if (listeners) {
this.events[eventName] = listeners.filter(listener => {
return listener !== listenerToRemove;
});
}
}
// Method to remove all listeners for a given event
removeAllListeners(eventName) {
// Delete the array of listeners for the specified event
delete this.events[eventName];
}
}
Above is a brief implementation. Now, let's see how we can use it:
// Create a new EventEmitter instance
const emitter = new EventEmitter();
// Add an event listener
emitter.on('greet', (name) => {
console.log(`Hello, ${name}!`);
});
// Trigger the 'greet' event
emitter.emit('greet', 'Alice'); // Output: Hello, Alice!
// Remove an event listener
const greetListener = (name) => {
console.log(`Hola, ${name}!`);
};
emitter.on('greet', greetListener);
emitter.off('greet', greetListener);
// Remove all listeners for 'greet' event
emitter.removeAllListeners('greet');
That's it! We've successfully implemented an EventEmitter and demonstrated its simple usage.
EventTarget
and EventEmitter
are both mechanisms for handling events. They share some similarities in usage and implementation but also have differences:
EventTarget
is the standard event interface in browsers, suitable for various web development scenarios.EventEmitter
is primarily used for event-driven programming in Node.js, but it can also be used in browsers.EventEmitter
offers additional functionalities such as one-time event listeners and error handling, making it more suitable for complex event handling scenarios.
In real-world applications, we can choose the appropriate event handling mechanism based on specific requirements and contexts.